2021.10.29
STAFF BLOG
スタッフブログ
TECHNICAL
テクログ
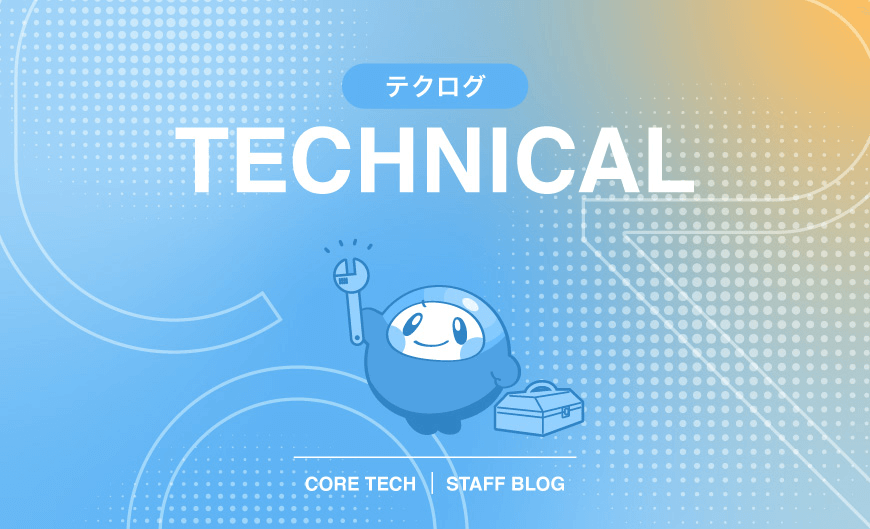
こんにちは!
今回は様々なPHPUnitで使用するアサーションやアノテーションについて紹介していきます!
各種用語について
アサーション とは
プログラミングにおいて、あるコードが実行される時に満たされるべき条件を記述して実行時にチェックする仕組み
アノテーションとは
メタデータを表す特別な構文のこと
PHPUnit などのアプリケーションでは、 この情報をもとに実行時の振る舞いを設定する
アサーションの種類
PHPUnitの公式ドキュメント(https://phpunit.readthedocs.io/ja/latest/assertions.html)からいくつかピックアップして紹介します!
assertEquals()
assertEquals(mixed $expected, mixed $actual[, string $message = ''])
2 つの変数
PHPUnit Docs »1. アサーション$expected
と$actual
が等しくない場合にエラー$message
を報告します。
<?php
use PHPUnit\Framework\TestCase;
class EqualsTest extends TestCase
{
public function testFailure()
{
$this->assertEquals(1, 0);
}
public function testFailure2()
{
$this->assertEquals('bar', 'baz');
}
public function testFailure3()
{
$this->assertEquals("foo\nbar\nbaz\n", "foo\nbah\nbaz\n");
}
}
$ phpunit EqualsTest
PHPUnit 7.0.0 by Sebastian Bergmann and contributors.
FFF
Time: 0 seconds, Memory: 5.25Mb
There were 3 failures:
1) EqualsTest::testFailure
Failed asserting that 0 matches expected 1.
/home/sb/EqualsTest.php:6
2) EqualsTest::testFailure2
Failed asserting that two strings are equal.
--- Expected
+++ Actual
@@ @@
-'bar'
+'baz'
/home/sb/EqualsTest.php:11
3) EqualsTest::testFailure3
Failed asserting that two strings are equal.
--- Expected
+++ Actual
@@ @@
'foo
-bar
+bah
baz
'
/home/sb/EqualsTest.php:16
FAILURES!
Tests: 3, Assertions: 3, Failures: 3.
assertSame()
assertSame(mixed $expected, mixed $actual[, string $message = ”])
2 つの変数
PHPUnit Docs »1. アサーション$expected
と$actual
が同じ型・同じ値でない場合にエラー$message
を報告します。
基本的にはassertEquals()を使用するより型まで比較するassertSame()を使用するほうが良さそうですね。
<?php
use PHPUnit\Framework\TestCase;
class SameTest extends TestCase
{
public function testFailure()
{
$this->assertSame('2204', 2204);
}
}
?>
$ phpunit SameTest
PHPUnit 7.0.0 by Sebastian Bergmann and contributors.
F
Time: 0 seconds, Memory: 5.00Mb
There was 1 failure:
1) SameTest::testFailure
Failed asserting that 2204 is identical to '2204'.
/home/sb/SameTest.php:6
FAILURES!
Tests: 1, Assertions: 1, Failures: 1.
assertRegExp()
assertRegExp(string $pattern, string $string[, string $message = ”])
PHPUnit Docs »1. アサーション
$string
が正規表現$pattern
にマッチしない場合にエラー$message
を報告します。
<?php
use PHPUnit\Framework\TestCase;
class RegExpTest extends TestCase
{
public function testFailure()
{
$this->assertRegExp('/foo/', 'bar');
}
}
?>
$ phpunit RegExpTest
PHPUnit 7.0.0 by Sebastian Bergmann and contributors.
F
Time: 0 seconds, Memory: 5.00Mb
There was 1 failure:
1) RegExpTest::testFailure
Failed asserting that 'bar' matches PCRE pattern "/foo/".
/home/sb/RegExpTest.php:6
FAILURES!
Tests: 1, Assertions: 1, Failures: 1.
assertArrayHasKey()
assertArrayHasKey(mixed $key, array $array[, string $message = ”])
PHPUnit Docs »1. アサーション
$array
にキー$key
が存在しない場合にエラー$message
を報告します。
<?php
use PHPUnit\Framework\TestCase;
class ArrayHasKeyTest extends TestCase
{
public function testFailure()
{
$this->assertArrayHasKey('foo', ['bar' => 'baz']);
}
}
$ phpunit ArrayHasKeyTest
PHPUnit 7.0.0 by Sebastian Bergmann and contributors.
F
Time: 0 seconds, Memory: 5.00Mb
There was 1 failure:
1) ArrayHasKeyTest::testFailure
Failed asserting that an array has the key 'foo'.
/home/sb/ArrayHasKeyTest.php:6
FAILURES!
Tests: 1, Assertions: 1, Failures: 1.
assertObjectHasAttribute()
assertObjectHasAttribute(string $attributeName, object $object[, string $message = ''])
PHPUnit Docs »1. アサーション
$object->attributeName
が存在しない場合にエラー$message
を報告します。
<?php
use PHPUnit\Framework\TestCase;
class ObjectHasAttributeTest extends TestCase
{
public function testFailure()
{
$this->assertObjectHasAttribute('foo', new stdClass);
}
}
?>
$ phpunit ObjectHasAttributeTest
PHPUnit 7.0.0 by Sebastian Bergmann and contributors.
F
Time: 0 seconds, Memory: 4.75Mb
There was 1 failure:
1) ObjectHasAttributeTest::testFailure
Failed asserting that object of class "stdClass" has attribute "foo".
/home/sb/ObjectHasAttributeTest.php:6
FAILURES!
Tests: 1, Assertions: 1, Failures: 1.
assertCount()
assertCount($expectedCount, $haystack[, string $message = ''])
PHPUnit Docs »1. アサーション
$haystack
の要素数が$expectedCount
でない場合にエラー$message
を報告します。
<?php
use PHPUnit\Framework\TestCase;
class CountTest extends TestCase
{
public function testFailure()
{
$this->assertCount(0, ['foo']);
}
}
$ phpunit CountTest
PHPUnit 7.0.0 by Sebastian Bergmann and contributors.
F
Time: 0 seconds, Memory: 4.75Mb
There was 1 failure:
1) CountTest::testFailure
Failed asserting that actual size 1 matches expected size 0.
/home/sb/CountTest.php:6
FAILURES!
Tests: 1, Assertions: 1, Failures: 1.
このような感じで様々な種類のテストができることが分かると思います!
テストケースに沿った適切なアサーションを選択していきたいですね!
アノテーションの種類
PHPUnitの公式ドキュメント(https://phpunit.readthedocs.io/ja/latest/assertions.html)からいくつかピックアップして紹介します!
@test
テストメソッド名の先頭に
PHPUnit Docs »2. アノテーションtest
をつけるかわりに、メソッドのドキュメンテーションブロックで@test
アノテーションを使ってそのメソッドがテストメソッドであることを指定することができます。
/**
* @test
*/
public function initialBalanceShouldBe0()
{
$this->assertSame(0, $this->ba->getBalance());
}
@group
あるテストを、ひとつあるいは複数のグループに属するものとすることができます。
PHPUnit Docs »2. アノテーション@group
アノテーションをこのように使用します。
use PHPUnit\Framework\TestCase;
class MyTest extends TestCase
{
/**
* @group specification
*/
public function testSomething()
{
}
/**
* @group regresssion
* @group bug2204
*/
public function testSomethingElse()
{
}
}
@covers
PHPUnit Docs »2. アノテーション
@covers
アノテーションをテストコードで使うと、 そのテストメソッドがどのメソッドをテストするのかを指定することができます。
/**
* @covers BankAccount::getBalance
*/
public function testBalanceIsInitiallyZero()
{
$this->assertSame(0, $this->ba->getBalance());
}